This article describes how to use Python to output textures in Substance 3D Designer. I would like to output the following test material.
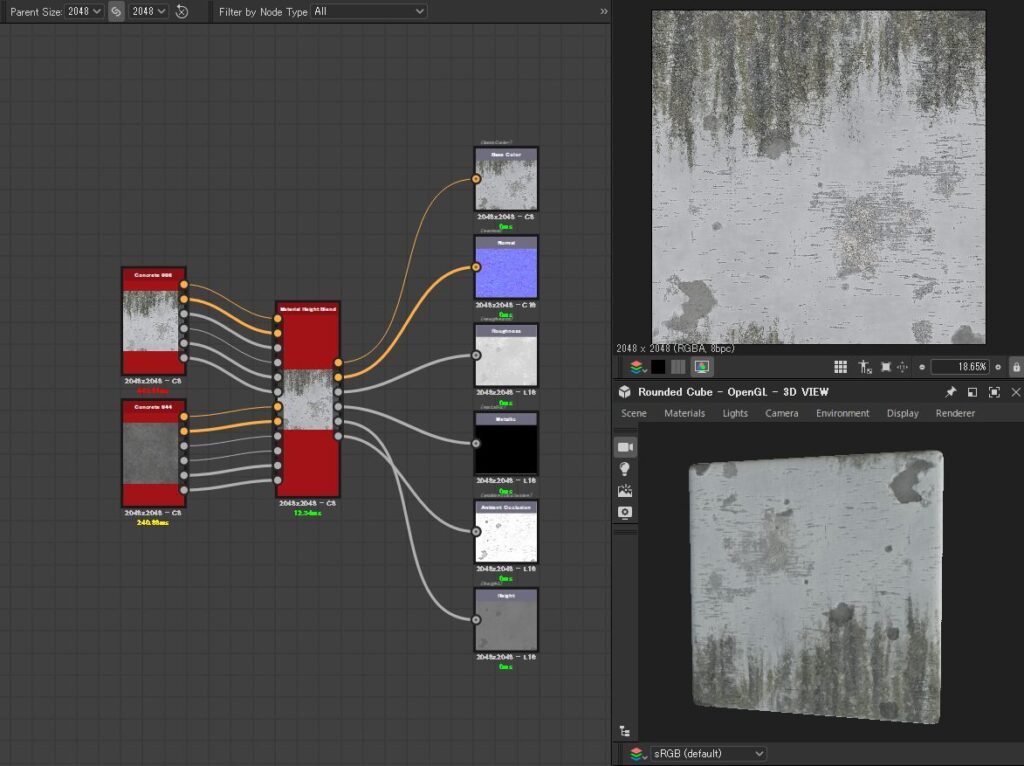
Contents
Output textures manually
First, let’s look at how to manually output textures.
Right-click on the graph and select Export outputs to output textures.
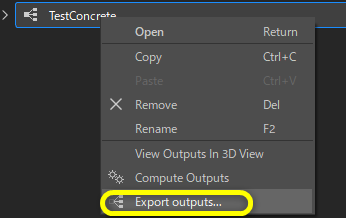
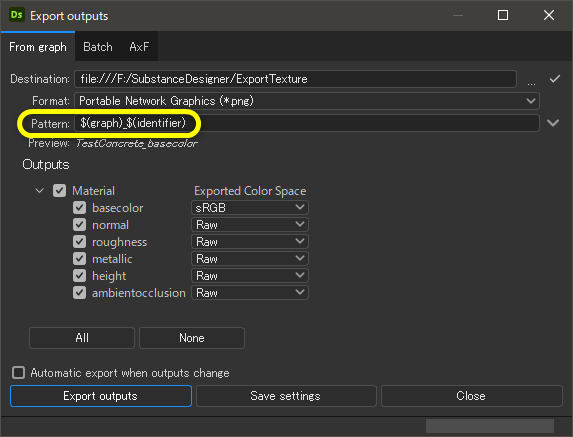
The output file name defaults to graph_name_identifier of the output node.
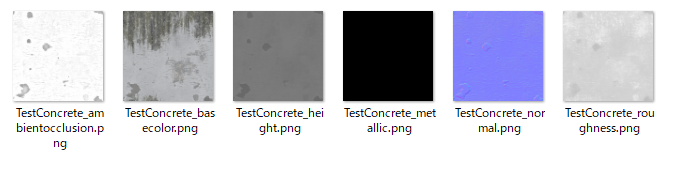
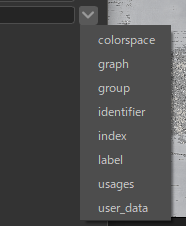
The file name pattern can be changed from the pull-down menu on the right to change which element is used.
Output textures in Python
For texture output in Python, the sd.tools.export.exportSDGraphOutputs function is provided. Let’s use this to output.
import sd
from sd.tools.export import exportSDGraphOutputs
context = sd.getContext()
app = context.getSDApplication()
ui_mgr = app.getQtForPythonUIMgr()
# Get current graph
graph = ui_mgr.getCurrentGraph()
export_path = "F:/SubstanceDesigner/ExportTexturePy"
exportSDGraphOutputs(graph, export_path, 'png')
Let’s run this code from the Python Editor.
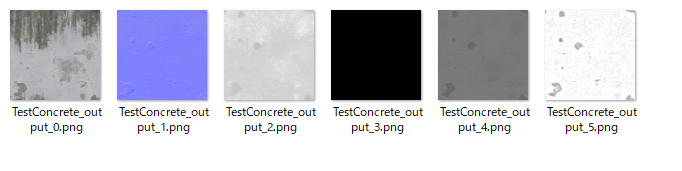
The output texture name appears to be graph_name_output_sequential_number.
I wonder why this is the case…
The file names seem to be conclusive with this naming convention.
The exportSDGraphOutputs function is described below.
Create your own texture output functions
Modify the sd.tools.export.exportSDGraphOutputs function in the library,
I will create my own texture output function to output textures, where the output file name is the graph name_identifier of the output node.
import sd
import os
from sd.api.sbs import sdsbscompgraph
from sd.api import sdproperty
from sd.api.apiexception import APIException
def export_texture(aSDGraph, aOutputDir = '', aFileExt = 'png'):
if not aSDGraph:
return False
if not issubclass(type(aSDGraph), sdsbscompgraph.SDSBSCompGraph):
return False
aSDGraph.compute()
graphIdentifier = aSDGraph.getIdentifier()
nodeIndex = -1
for sdNode in aSDGraph.getOutputNodes():
nodeIndex = nodeIndex + 1
nodeDefinition = sdNode.getDefinition()
outputProperties = nodeDefinition.getProperties(sdproperty.SDPropertyCategory.Output)
for outputProperty in outputProperties:
propertyValue = sdNode.getPropertyValue(outputProperty)
propertyTexture = propertyValue.get()
if not propertyTexture:
continue
fileExt = aFileExt
identifier = outputProperty.getId()
fileName = str(graphIdentifier) + '_' + identifier + '.'+ str(fileExt)
textureFileName = os.path.abspath(os.path.join(aOutputDir, fileName))
try:
propertyTexture.save(textureFileName)
except APIException:
print('Fail to save texture %s' % textureFileName)
return True
context = sd.getContext()
app = context.getSDApplication()
ui_mgr = app.getQtForPythonUIMgr()
graph = ui_mgr.getCurrentGraph()
export_path = "F:/SubstanceDesigner/ExportTexturePy"
export_texture(graph, export_path, 'png')
The output with this code will have the following file name.
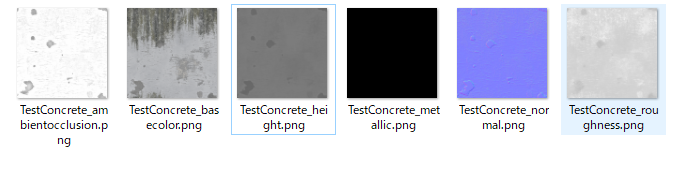
You did it!
You can create a function that outputs with your own naming convention and make it into a library.
Reference Articles
The following article written by Kamosoba shows an example of implementing your own function in export.py.
This is a very helpful way to output animated textures in scripts.
(Install path) /resources/python/sd/tools/export.py